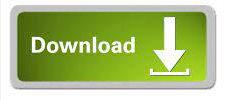
There are times when ensuring your original list is not mutated is beneficial, and conversely there are times when performance concerns might mean you don’t want to create a new copy of the list each time you add to it. For other collections, like ArrayList, you’ll be able to choose either approach. For some collection types, there won’t even be an option. There’s probably not a single answer here which is best will depend on your use case.
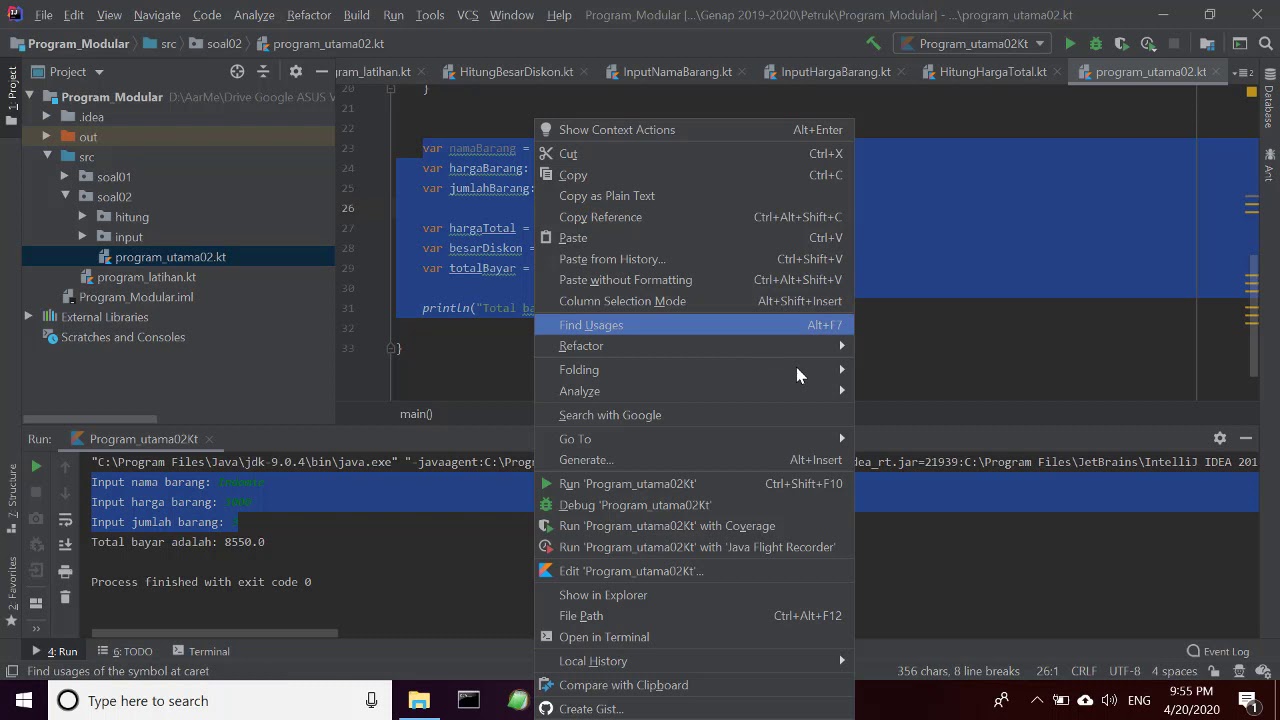
Whereas if you explicitly have a MutableList then += can do the more performant adding of the item without creating a whole new list.

This is because a List type doesn’t indicate it is mutable. When the declared type is a List, then calling += will result in creating a brand new list and copying the old one into it. Why? As above, the type you’ve declared the list as (rather than the implementation itself) is the determining factor in what += will actually do when you use it. Calling += adds the element to existing list.Calling += copies the whole list into a new list.Under the hood, in each case above we are dealing with ArrayLists. option 1 var list : List = ArrayList () list += 1 // option 2 var list : List = mutableListOf () list += 1 // option 3 val list = mutableListOf () list += 1 One way we can do that is to reassign the original list var to the new list which is returned each time (note because we are reassigning the variable, we need to switch from val to var): To fix this, we need to stop ignoring the returned list. We call foo + 2 and are given another new list back, which we ignore again. When we call foo + 1 we are given a new list back that we ignore.
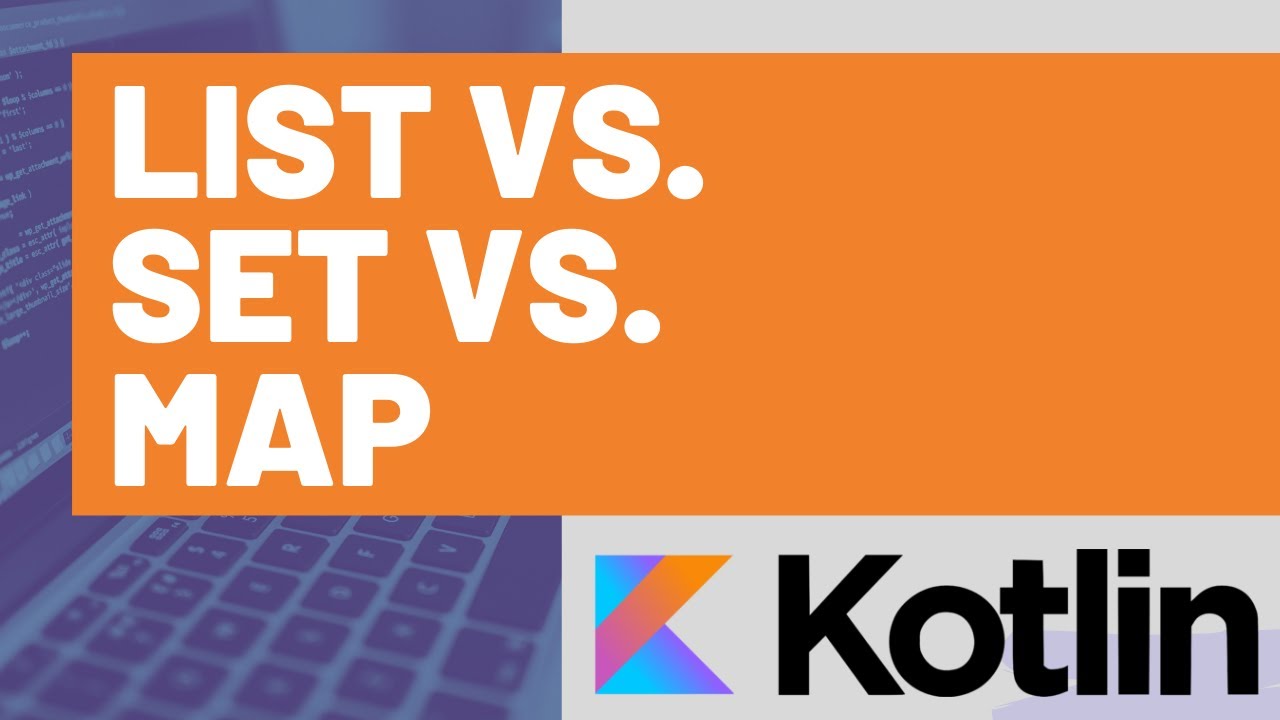

So this now explains why, in our original example, we were left looking at an empty list. Here’s the important thing to remember: it doesn’t modify the original list.
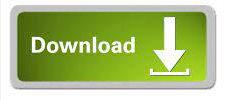